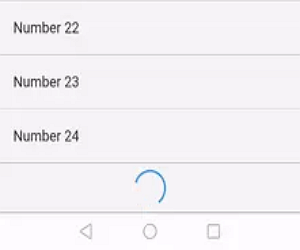
Dynamic List View
A list component that can refreshes and adds more data for Flutter App. 🚀
Installation
Add this to your package’s pubspec.yaml file:
dependencies:
dynamic_list_view: ^0.1.9
Usage example
import 'package:dynamic_list_view/DynamicListView.dart';
import 'package:flutter/material.dart';
import 'dart:async';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Container(
child: DynamicListView.build(
itemBuilder: _itemBuilder,
dataRequester: _dataRequester,
initRequester: _initRequester,
),
),
),
);
}
Future<List> _initRequester() async {
return Future.value(List.generate(15, (i) => i));
}
Future<List> _dataRequester() async {
return Future.delayed(Duration(seconds: 2), () {
return List.generate(10, (i) => 15 + i);
});
}
Function _itemBuilder = (List dataList, BuildContext context, int index) {
String title = dataList[index].toString();
return ListTile(title: Text("Number $title"));
};
}
Contribute
We would ❤️ to see your contribution!
License
Distributed under the MIT license. See
for more information.LICENSE
About
Created by Shusheng.
Source Code
Please Visit Flutter Dynamic List View Source Code at GitHub
Leave a Reply