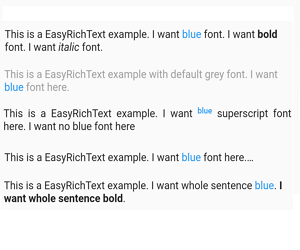
easy_rich_text
The EasyRichText widget provides a easy way to use RichText when you want to use specific style for specific word pattern. This widget would be useful when you want to apply RichText to text get from a query.
This widget split string into multiple TextSpan by defining a List of EasyRichTextPattern();
The EasyRichTextPattern is a class defines the text pattern you want to format.
Getting Started
Installing:
dependencies:
easy_rich_text: '^0.2.2'
Simple example:
String str1 = "This is a EasyRichText example. I want blue font. I want bold font. I want italic font. ";
EasyRichText(
str1,
patternList: [
EasyRichTextPattern(
targetString: 'blue',
style: TextStyle(color: Colors.blue),
),
EasyRichTextPattern(
targetString: 'bold',
style: TextStyle(fontWeight: FontWeight.bold),
),
EasyRichTextPattern(
targetString: 'italic',
style: TextStyle(fontStyle: FontStyle.italic),
),
],
),
Default Style:
String str2 = "This is a EasyRichText example with default grey font. I want blue font here.";
EasyRichText(
str2,
defaultStyle: TextStyle(color: Colors.grey),
patternList: [
EasyRichTextPattern(
targetString: 'blue',
style: TextStyle(color: Colors.blue),
),
EasyRichTextPattern(
targetString: 'bold',
style: TextStyle(fontWeight: FontWeight.bold),
),
],
),
superscript and subscript.
String str3 = "This is a EasyRichText example. I want blue superscript font here. I want no blue font here";
EasyRichText(
str3,
patternList: [
EasyRichTextPattern(
targetString: 'blue',
stringBeforeTarget: 'want',
style: TextStyle(color: Colors.blue),
superScript: true),
],
textAlign: TextAlign.justify,
),
All RichText properties accessible: textAlign, maxLines, overflow, etc.
String str4 = "This is a EasyRichText example. I want blue font here. TextOverflow.ellipsis, TextAlign.justify, maxLines: 1";
EasyRichText(
str4,
patternList: [
EasyRichTextPattern(
targetString: 'blue',
stringBeforeTarget: 'want',
style: TextStyle(color: Colors.blue),
),
],
textAlign: TextAlign.justify,
maxLines: 1,
overflow: TextOverflow.ellipsis,
),
Known issues
Conflict when one target string is included in another target string
String str6 = "This is a EasyRichText example. I want whole sentence blue. I want whole sentence bold.";
EasyRichText(
str6,
patternList: [
EasyRichTextPattern(
targetString: 'blue',
style: TextStyle(color: Colors.blue),
),
EasyRichTextPattern(
targetString: 'I want whole sentence blue',
style: TextStyle(fontWeight: FontWeight.bold),
),
EasyRichTextPattern(
targetString: 'I want whole sentence bold',
style: TextStyle(fontWeight: FontWeight.bold),
),
],
),
not all characters support superscript and subscript
Characters do not support superscript: q z C F Q S X Y Z.
Only these characters support subscript: e h i j k l m n o p r s t u v x.
Source Code
Please Visit Flutter RichText With Multiple Styles Source at GitHub