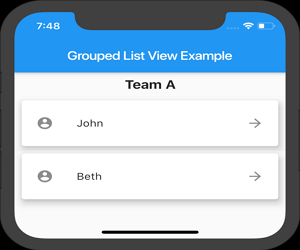
Grouped list package for Flutter
A Flutter ListView Grouped Item in which list items can be grouped to sections.
Getting Started
Add the package to your pubspec.yaml:
grouped_list: ^1.3.1
In your dart file, import the library:
import 'package:grouped_list/grouped_list.dart';
Instead of using a ListView
create a GroupedListView
Widget:
GroupedListView(
elements: _elements,
groupBy: (element) => element['group'],
groupSeparatorBuilder: _buildGroupSeparator,
itemBuilder: (context, element) => Text(element['name']),
order: GroupedListOrder.ASC,
),
You can also use most fields from the `ListView.builder` constructor.
Required Parameters:
- `elements`: A list of the data you want to display in the list.
- `groupBy`: Function which maps an element to its grouped value.
- `itemBuilder`: Function which returns an Widget which defines the item.
- `groupSeparator`: Function which returns an Widget which defines the section separator.
Widget _buildGroupSeparator(dynamic groupByValue) { return Text('$groupByValue'); }
The parameter
groupByValue
has the return type of the definedgroupBy
function.
Optional Parameters:
order
: By default it’s GroupedListOrder.ASC. Change to GroupedListOrder.DESC for reversing the group sorting.separator
: A Widget which defines a separator between items inside a section.sort
: A bool which defines if the passed data should be sorted by the widget. By default it’s true.
Notice:
- The item builder functions only creates the actual list items. For seperator items use the
separator
parameter. - Other than the
itemBuilder
function of theListView.builder
constructor the function provides the specific element instead of the index as parameter. - The elements need to be sorted according to the
groupBy
return value. The Widgets sorts the elements by default. Disable the sorting only if your list is sorted beforehand.
Source Code
Please Visit Flutter ListView Group Source Code at GitHub