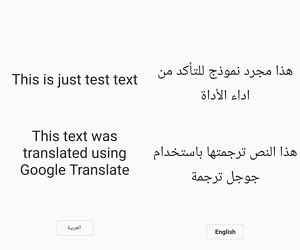
localize_and_translate
Flutter localization abstract, Really simple
Show some :heart: and star the repo
Screenshots
Tutorial
Video
Methods
Method | Job | ||
---|---|---|---|
init() |
initialize things, before runApp() | ||
translate('word') |
word translation | ||
translate('word',{"key":"value"}) |
word translation with replacement arguments | ||
<!– | googleTranslate('word', from: 'en', to: 'ar') |
google translate | –> |
setNewLanguage(context,newLanguage:'en',restart: true, remember: true,) |
change language | ||
isDirectionRTL() |
is Direction RTL check | ||
currentLanguage |
Active language code | ||
locale |
Active Locale | ||
locals() |
Locales list | ||
delegates |
Localization Delegates |
Installation
-
add
.json
translation files as assets -
For example :
'assets/langs/ar.json'
|'assets/langs/en.json'
-
structure should look like
{
"appTitle" : "تطبيق",
"textArea" : "Thisi is just a test text"
}
- define them as assets in pubspec.yaml
flutter:
assets:
- assets/langs/en.json
- assets/langs/ar.json
Initialization
- Add imports to main.dart
- Make
main()
async
and do the following - Ensure flutter activated
WidgetsFlutterBinding.ensureInitialized()
- Define languages list
LIST_OF_LANGS
- Define assets directory
LANGS_DIR
- Initialize
await translator.init();
- Inside
runApp()
wrap entry class withLocalizedApp()
- Note : make sure you define it’s child into different place "NOT INSIDE"
import 'package:flutter/material.dart';
import 'package:localize_and_translate/localize_and_translate.dart';
main() async {
// if your flutter > 1.7.8 : ensure flutter activated
WidgetsFlutterBinding.ensureInitialized();
LIST_OF_LANGS = ['ar', 'en']; // define languages
LANGS_DIR = 'assets/langs/'; // define directory
await translator.init(); // intialize
runApp(
LocalizedApp(
child: MyApp(),
),
);
}
LocalizedApp()
child example ->MaterialApp()
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Home(),
localizationsDelegates: translator.delegates, // Android + iOS Delegates
locale: translator.locale, // Active locale
supportedLocales: translator.locals(), // Locals list
);
}
}
Usage
- use
translate("appTitle")
- use
googleTranslate("test", from: 'en', to: 'ar')
- use `setNewLanguage(context, newLanguage: ‘ar’, remember: true, restart: true);
class Home extends StatefulWidget {
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
String testText =
translator.currentLanguage == 'ar' ? 'جار الترجمة' : 'Translating..';
@override
void initState() {
super.initState();
Future.delayed(Duration.zero, () async {
testText = await translator.googleTranslate(
testText,
from: 'en',
to: translator.currentLanguage,
);
setState(() {});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
drawer: Drawer(),
appBar: AppBar(
title: Text(translator.translate('appTitle')),
// centerTitle: true,
),
body: Container(
width: double.infinity,
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: <Widget>[
SizedBox(height: 50),
Text(
translator.translate('textArea'),
textAlign: TextAlign.center,
style: TextStyle(fontSize: 35),
),
Text(
testText,
textAlign: TextAlign.center,
style: TextStyle(fontSize: 35),
),
OutlineButton(
onPressed: () {
translator.setNewLanguage(
context,
newLanguage: translator.currentLanguage == 'ar' ? 'en' : 'ar',
remember: true,
restart: true,
);
},
child: Text(translator.translate('buttonTitle')),
),
],
),
),
);
}
}
Contributions
translate()
accept keys : by mo-ah-dawood- Change template from plugin to package : by mo-ah-dawood
Known Issues
- Lowest Flutter Version (1.12.13)
Project Created & Maintained By
Software Engineer | In :heart: with Flutter
Donate
If you found this project helpful or you learned something from the source code and want to thank me, consider buying me a cup of :coffee:
Source Code
Please Visit Flutter Localize and Translate Source Code at GitHub