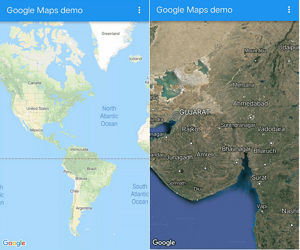
Flutter Google Map Example
A Flutter application demonstrate google map.
Preview
Listener and Marker | Compass | MapTypes |
---|---|---|
![]() |
![]() |
![]() |
Plugin
google_maps_flutter from flutter team.
Usage
To use this plugin, add
google_maps_flutter: latest_version
as a dependency in your pubspec.yaml file.
Getting Started
Get an API key at https://cloud.google.com/maps-platform/.
Android
Specify your API key in the application manifest android/app/src/main/AndroidManifest.xml
:
<manifest ...
<application ...
<meta-data android:name="com.google.android.geo.API_KEY"
android:value="YOUR KEY HERE"/>
iOS
Specify your API key in the application delegate ios/Runner/AppDelegate.m
:
Objective-C
#include "AppDelegate.h"
#include "GeneratedPluginRegistrant.h"
#import "GoogleMaps/GoogleMaps.h"
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[GMSServices provideAPIKey:@"YOUR KEY HERE"];
[GeneratedPluginRegistrant registerWithRegistry:self];
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
@end
Swift
import UIKit
import Flutter
import GoogleMaps
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
GMSServices.provideAPIKey("YOUR_API_KEY")
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
}
Opt-in to the embedded views preview by adding a boolean property to the app’s Info.plist
file
with the key io.flutter.embedded_views_preview
and the value YES
.
Both
You can now add a GoogleMap
widget to your widget tree.
The map view can be controlled with the GoogleMapController
that is passed to
the GoogleMap
‘s onMapCreated
callback.
Contribute
- Fork the the project
- Create your feature branch (git checkout -b my-new-feature)
- Make required changes and commit (git commit -am ‘Add some feature’)
- Push to the branch (git push origin my-new-feature)
- Create new Pull Request
Donate
If you found this project helpful or you learned something from the source code and want to thank me, consider buying me a cup of :coffee:
- PayPal
- Google Pay (bhavikmakwana43@okhdfcbank)
Source Code
Please Visit Flutter Google Map Example Source Code at GitHub